Hello, Now I want share how to use Qt, previously is using Wxwidget and now I want share using Qt (especially Qt5). Form login will look like this:
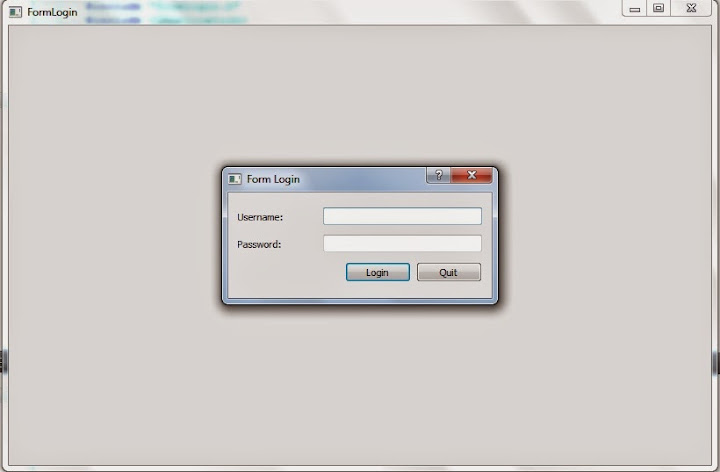
Okay to create the application like the picture above, follow this instructions:
First open your Qt creator and create new Project (based Qt widgets application) and give the name of the application with the name of the “FormLogin”.
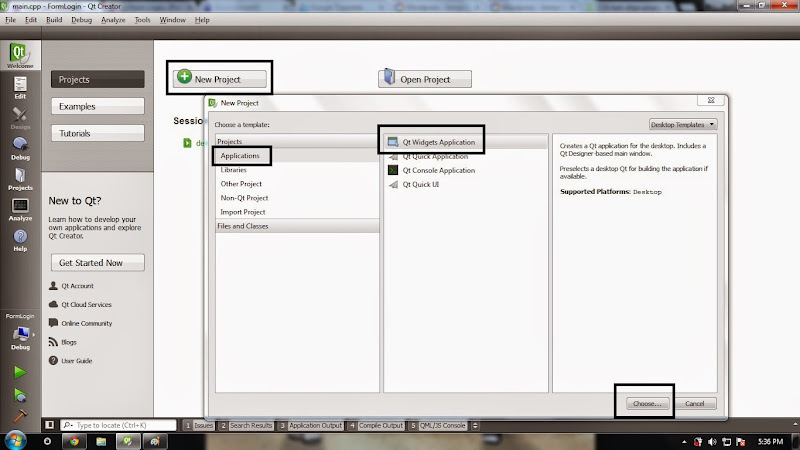
After you create the project, the structure FormLogin application will look like this:
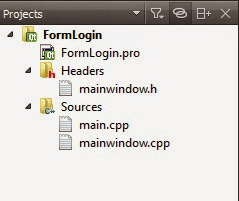
After that, right click the project and select add new. Then choose C++ header file and give the name “formlogin”.
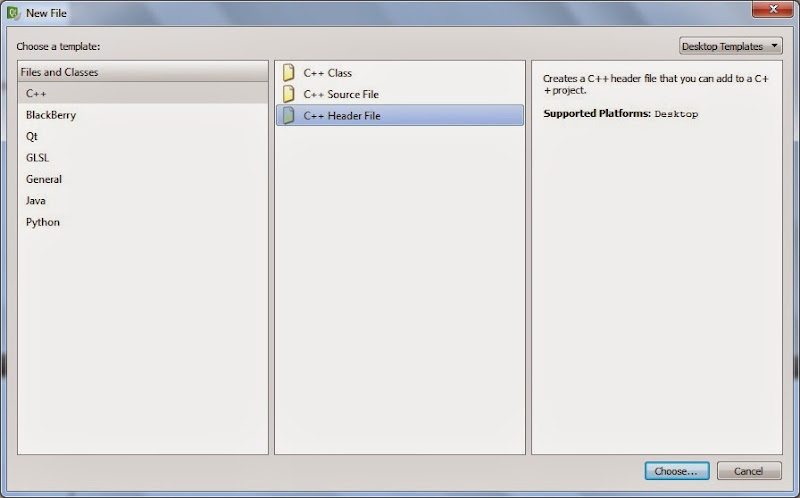
Repeat the above steps, but in popup window new file, select “C++ source file” and give the name “formlogin”. Then you will get a directory structure like this:

And now we must coding :D. First open your formlogin.h and insert this code:
#ifndef FORMLOGIN_H #define FORMLOGIN_H #include <QDialog> #include <QVBoxLayout> #include <QHBoxLayout> #include <QLabel> #include <QLineEdit> #include <QPushButton> #include <QMessageBox> class FormLogin : public QDialog { Q_OBJECT public: FormLogin(QWidget* parent = 0); ~FormLogin(); private slots: void OnQuit(); void OnLogin(); private: void reject(); QLabel* userLabel; QLabel* passLabel; QLineEdit* userLineEdit; QLineEdit* passLineEdit; QPushButton* loginButton; QPushButton* quitButton; }; #endif // FORMLOGIN_H
Then add this code on formlogin.cpp:
#include "formlogin.h" FormLogin::FormLogin(QWidget* parent) : QDialog(parent) { setFixedSize(300, 120); setWindowTitle("Form Login"); setModal(true); setAttribute(Qt::WA_DeleteOnClose); userLabel = new QLabel("Username:"); passLabel = new QLabel("Password:"); userLineEdit = new QLineEdit(); passLineEdit = new QLineEdit(); passLineEdit->setEchoMode(QLineEdit::Password); loginButton = new QPushButton("Login"); quitButton = new QPushButton("Quit"); QVBoxLayout* vbox = new QVBoxLayout(this); QHBoxLayout* hbox1 = new QHBoxLayout(); QHBoxLayout* hbox2 = new QHBoxLayout(); QHBoxLayout* hbox3 = new QHBoxLayout(); hbox1->addWidget(userLabel, 1); hbox1->addWidget(userLineEdit, 2); hbox2->addWidget(passLabel, 1); hbox2->addWidget(passLineEdit, 2); hbox3->addWidget(loginButton, 1, Qt::AlignRight); hbox3->addWidget(quitButton, 0, Qt::AlignRight); vbox->addSpacing(1); vbox->addLayout(hbox1); vbox->addLayout(hbox2); vbox->addLayout(hbox3); connect(quitButton, SIGNAL(clicked()), this, SLOT(OnQuit())); connect(loginButton, SIGNAL(clicked()), this, SLOT(OnLogin())); } void FormLogin::reject() { OnQuit(); } void FormLogin::OnQuit() { this->close(); parentWidget()->close(); } void FormLogin::OnLogin() { QString username = userLineEdit->text(); QString password = passLineEdit->text(); // Checking if username or password is empty if (username.isEmpty() || password.isEmpty()) QMessageBox::information(this, tr("Peringatan!"), "Username atau password tidak boleh kosong"); else this->destroy(); } FormLogin::~FormLogin() {}
Explanations:
- Line 7. Setfixed is used to fixed the size of the window, so the window can’t resize and not have maximaze button.
- Line 8. This function is used to create the modal dialog, so if user click the main window, the form login will blink and sound an alert sound
- Line 9. Setting Qt::WA_DeleteOnClose means qt can delete anytime after you call close()
- Line 15. To display platform-dependent password mask characters instead of the characters actually entered.
- Line 28-29. Is used to make the button is positioned on the right, look login and quit button from above picture
- Line 31. This function is to add space between widget
- Line 40-43. This function will be called when the user clicks the close button and call FormLogin::OnQuit function
- Line 45-49. THis function will be called when the user clicks the quit button. Then this function will delete/close form login window and main window.
- Line 53-54. This function to get text from line edit
- Line 57-58. This code is if user click login button and the username and password is empty, user will get warning prompt
- Line 60 is used if user success login and form login will close
Now open mainwindow.h and write this code:
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> class MainWindow : public QMainWindow { Q_OBJECT public: MainWindow(); ~MainWindow(); }; #endif // MAINWINDOW_H
And this is the mainwindow.cpp source:
#include "mainwindow.h" MainWindow::MainWindow() : QMainWindow() { setMinimumSize(800, 600); } MainWindow::~MainWindow() {}
And the last is implement formlogin and mainwindow on main.cpp:
#include "mainwindow.h" #include "formlogin.h" #include <QApplication> int main(int argc, char *argv[]) { QApplication a(argc, argv); MainWindow* mainWindow = new MainWindow(); FormLogin* formLogin = new FormLogin(mainWindow); formLogin->show(); mainWindow->show(); return a.exec(); }
Explanation:
- Line 8 is to create pointer of mainwindow
- Line 9 is to instance formlogin with the parent window is mainwidow. Look the formlogin.h line 17. This code is to add constructor with parent (default parent is null/0), and from formlogin.cpp line 3-4 is to call constructor QDialog with parent QDialog(parent).
-
Now run the project, if no error you will get form login application like the first picture on this post.
Source code: http://downloads.ziddu.com/download/24288305/FormLogin.7z.html
Thank you for any other informative web site. The place else could I am getting that kind of information written in such an ideal method? I’ve a mission that I am just now working on, and I have been at the look out for such information.
Hello, the whole thing is going fine here and ofcourse every one is sharing information, that’s actually excellent, keep up writing.
Good response in return of this matter with firm arguments and describing the whole thing about that.
What’s Taking place i’m new to this, I stumbled upon this I have discovered It positively useful and it has helped me out loads. I’m hoping to contribute & help different users like its aided me. Good job.
Wow, that’s what I was looking for, what a information! existing here at this web site, thanks admin of this web page.
wonderful points altogether, you simply gained a logo new reader. What might you suggest in regards to your submit that you simply made a few days in the past? Any positive?
obviously like your web-site but you have to check the spelling on quite a few of your posts. A number of them are rife with spelling problems and I in finding it very troublesome to inform the reality on the other hand I’ll surely come again again.
Superb blog! Do you have any tips for aspiring writers? I’m planning to start my own website soon but I’m a little lost on everything. Would you recommend starting with a free platform like WordPress or go for a paid option? There are so many choices out there that I’m completely confused .. Any suggestions? Cheers!
Yes you can using wordpress like me.. đŸ™‚
Actually no matter if someone doesn’t be aware of after that its up to other viewers that they will assist, so here it occurs.
There is certainly a great deal to learn about this subject. I like all of the points you’ve made.
There’s certainly a great deal to learn about this subject. I like all of the points you’ve made.
You really make it seem so easy with your presentation however I in finding this matter to be really something which I believe I would by no means understand. It kind of feels too complicated and very broad for me. I am taking a look forward on your next post, I will attempt to get the hold of it!
Good post. I absolutely love this site. Keep writing!
I relish, lead to I discovered exactly what I was having a look for. You have ended my four day lengthy hunt! God Bless you man. Have a great day. Bye
What’s up, after reading this awesome piece of writing i am also glad to share my familiarity here with mates.
Everyone loves what you guys are usually up too. This kind of clever work and reporting! Keep up the terrific works guys I’ve you guys to blogroll.
What’s up friends, how is all, and what you would like to say regarding this piece of writing, in my view its in fact amazing designed for me.
This is a great tip particularly to those fresh to the blogosphere. Short but very accurate information… Thanks for sharing this one. A must read article!
I read this paragraph completely regarding the difference of most up-to-date and previous technologies, it’s remarkable article.
What a stuff of un-ambiguity and preserveness of precious knowledge on the topic of unpredicted emotions.
Usually I do not learn post on blogs, however I wish to say that this write-up very pressured me to check out and do so! Your writing taste has been surprised me. Thank you, very great post.
Thanks for sharing your info. I really appreciate your efforts and I am waiting for your further post thank you once again.
Great weblog right here! Additionally your web site a lot up very fast! What host are you the usage of? Can I get your affiliate hyperlink in your host? I want my website loaded up as fast as yours lol
I am in fact thankful to the holder of this web page who has shared this great paragraph at at this place.
naturally like your web site however you have to test the spelling on quite a few of your posts. A number of them are rife with spelling issues and I to find it very troublesome to inform the reality nevertheless I’ll surely come back again.
Hi, its nice article on the topic of media print, we all be familiar with media is a great source of information.
Hi there, its pleasant post concerning media print, we all be aware of media is a impressive source of data.
May I just say what a relief to find an individual who actually knows what they are talking about over the internet. You definitely realize how to bring an issue to light and make it important. A lot more people need to look at this and understand this side of the story. I can’t believe you are not more popular since you definitely have the gift.
Everything is very open with a clear clarification of the challenges. It was really informative. Your website is very useful. Many thanks for sharing!
I’m pretty pleased to discover this web site. I wanted to thank you for ones time due to this wonderful read!! I definitely liked every bit of it and i also have you book marked to check out new things in your site.
I’ve read a few good stuff here. Certainly value bookmarking for revisiting. I wonder how much effort you set to make one of these great informative website.
Hurrah! Finally I got a webpage from where I be able to really take helpful information concerning my study and knowledge.
I’m not certain where you’re getting your information, however great topic. I must spend some time learning more or working out more. Thank you for magnificent info I was in search of this info for my mission.
It’s remarkable for me to have a web site, which is good for my knowledge. thanks admin
Spot on with this write-up, I actually think this web site needs a great deal more attention. I’ll probably be back again to read through more, thanks for the information!
Appreciating the time and energy you put into your blog and detailed information you offer. It’s great to come across a blog every once in a while that isn’t the same unwanted rehashed information. Fantastic read! I’ve saved your site and I’m adding your RSS feeds to my Google account.
I got this site from my friend who shared with me about this website and now this time I am browsing this web site and reading very informative articles here.
I drop a comment each time I appreciate a post on a blog or if I have something to valuable to contribute to the discussion. Usually it is caused by the passion communicated in the post I looked at. And on this post Qt Form Login. (Parent Window is Disabled After Child Window Login Success) . I was excited enough to drop a thought đŸ˜‰ I actually do have some questions for you if it’s okay. Is it only me or do a few of these comments come across as if they are left by brain dead visitors? đŸ˜› And, if you are posting on other social sites, I’d like to keep up with everything new you have to post. Could you list all of all your communal sites like your linkedin profile, Facebook page or twitter feed?
Very good post! We will be linking to this great post on our site. Keep up the great writing.
May I simply say what a relief to find an individual who truly knows what they’re discussing on the web. You actually realize how to bring an issue to light and make it important. More and more people have to check this out and understand this side of the story. I was surprised you are not more popular since you surely possess the gift.
What’s up, I want to subscribe for this webpage to obtain most up-to-date updates, therefore where can i do it please assist.
Hi there every one, here every one is sharing these experience, therefore it’s good to read this blog, and I used to go to see this website daily.
Its not my first time to pay a visit this site, i am visiting this web page dailly and obtain good information from here all the time.
Hello to all, it’s in fact a good for me to pay a quick visit this web site, it includes valuable Information.
Your style is so unique in comparison to other folks I’ve read stuff from. Thank you for posting when you have the opportunity, Guess I will just bookmark this blog.
Very good article. I absolutely love this site. Keep writing!
This information is worth everyone’s attention. How can I find out more?
hello!,I really like your writing so so much! percentage we be in contact extra about your article on AOL? I need an expert on this house to unravel my problem. May be that’s you! Having a look ahead to see you.
Piece of writing writing is also a excitement, if you be acquainted with afterward you can write if not it is complicated to write.
Right here is the perfect web site for anyone who would like to understand this topic. You understand so much its almost hard to argue with you (not that I actually will need to…HaHa). You certainly put a brand new spin on a topic that has been discussed for decades. Wonderful stuff, just wonderful!
For latest news you have to pay a visit world wide web and on internet I found this website as a best website for hottest updates.
This article will help the internet people for building up new blog or even a weblog from start to end.
If you are going for best contents like me, simply visit this website everyday because it provides quality contents, thanks
Excellent post. I’m going through some of these issues as well..
I like the valuable info you supply for your articles. I will bookmark your weblog and check once more right here regularly. I am slightly sure I will be informed a lot of new stuff right right here! Good luck for the next!
Hello every one, here every one is sharing such knowledge, therefore it’s nice to read this weblog, and I used to pay a quick visit this weblog every day.
Wow, that’s what I was looking for, what a data! existing here at this blog, thanks admin of this website.
My partner and I stumbled over here from a different website and thought I might as well check things out. I like what I see so i am just following you. Look forward to checking out your web page repeatedly.
Hi there I am so happy I found your site, I really found you by mistake, while I was searching on Google for something else, Anyhow I am here now and would just like to say thank you for a remarkable post and a all round entertaining blog (I also love the theme/design), I don’t have time to read through it all at the minute but I have book-marked it and also included your RSS feeds, so when I have time I will be back to read more, Please do keep up the great work.
Wonderful web site. Lots of useful information here. I am sending it to several buddies ans additionally sharing in delicious. And of course, thank you to your sweat!
Greetings I am so grateful I found your weblog, I really found you by mistake, while I was searching on Google for something else, Nonetheless I am here now and would just like to say thanks a lot for a incredible post and a all round entertaining blog (I also love the theme/design), I don’t have time to read through it all at the moment but I have book-marked it and also included your RSS feeds, so when I have time I will be back to read a great deal more, Please do keep up the fantastic job.
That is a great tip particularly to those new to the blogosphere. Simple but very accurate info… Many thanks for sharing this one. A must read post!
Good info. Lucky me I came across your website by accident (stumbleupon). I have bookmarked it for later!
What’s up, constantly i used to check website posts here early in the morning, for the reason that i like to find out more and more.
It’s an remarkable post designed for all the web people; they will obtain benefit from it I am sure.
I don’t know whether it’s just me or if perhaps everyone else experiencing issues with your website. It appears like some of the written text within your content are running off the screen. Can someone else please provide feedback and let me know if this is happening to them too? This might be a problem with my internet browser because I’ve had this happen before. Cheers
It’s genuinely very complex in this busy life to listen news on TV, therefore I only use web for that reason, and get the newest information.
Hello There. I found your weblog the usage of msn. This is a really neatly written article. I will be sure to bookmark it and return to learn more of your helpful info. Thank you for the post. I’ll certainly return.
Thanks for sharing such a pleasant opinion, paragraph is good, thats why i have read it entirely
What i don’t understood is in fact how you are not actually a lot more neatly-preferred than you may be right now. You’re very intelligent. You recognize thus significantly when it comes to this topic, produced me personally imagine it from numerous various angles. Its like women and men don’t seem to be fascinated unless it’s something to do with Woman gaga! Your personal stuffs nice. Always care for it up!
Right now it looks like Expression Engine is the best blogging platform available right now. (from what I’ve read) Is that what you are using on your blog?
I don’t even know how I stopped up here, however I believed this submit was once good. I don’t recognize who you’re but certainly you are going to a well-known blogger if you aren’t already. Cheers!
What’s up, I would like to subscribe for this blog to get hottest updates, so where can i do it please help.